Amazon Web Service Simple Email Service ( SES ) is a popular and considerably cheap service to send out bulk transactional & personalized promotional emails. However, when it comes to the Template-based emails which help to bind values and send HTML email, the most frustrating part is updating the template and testing them.
Problems:
1. Creating a valid JSON file by escaping the HTML Email Text property
2. Updating the templated HTML file ( manual command-line task )
3. Testing the templated emails
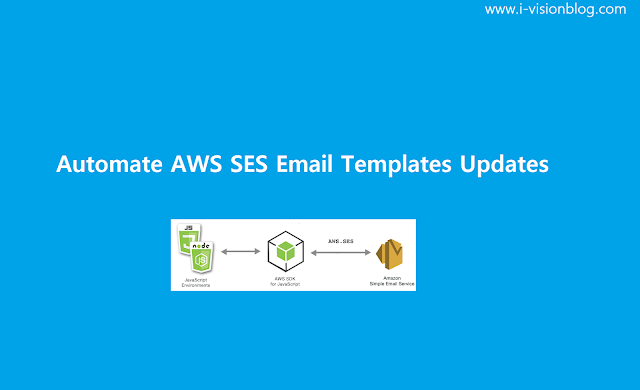 |
How to automate AWS SES E-mail Template Update |
Solution:
I've come up with a normal update script written in Nodejs language with the help of AWS SDK that can help in scaling email template updates faster.
Usage:
> node template-creater.js emailtemplate.html emailtemplate.json false emailtemplate-unique-name "Subject for the email"
This will automatically accept the templated HTML file and creates a valid JSON file and tries updating/creating templates in the AWS SES console based on configuration or command-line argument values.
1st Argument => Templated HTML File |
2nd Argument => Name of the auto-generated template json file |
3rd Argument => Update or Create template ( boolean ) |
4th Argument => Unique template name |
5th Argument => Subject of the email |
|
Final Words:
Feel free to use the script ( No license Restrictions for personal/commercial projects and No Warranty from Author ) and for bugs/hugs do comment below. Share is care.